In today’s fast-paced world, optimizing your code for better performance is crucial. With users expecting instant results, every millisecond counts. In this blog post, we’ll discuss 21 ways to optimize your code for improved efficiency and overall performance. Let’s dive in!
Achieve Peak Performance with These 21 Code Optimization Techniques
1. Minimize use of global variables
Global variables can make your code harder to maintain and debug, as well as reduce performance. By minimizing their use and relying on local variables instead, you’ll reduce the chance of conflicts and improve overall code execution speed. Consider using functions or classes to encapsulate data and logic, making it more modular and easier to manage.
For instance, instead of using global variables:
global_var = 0
def function():
global global_var
global_var += 1
Use local variables and pass them as arguments:
def function(local_var):
local_var += 1
return local_var
2. Use efficient data structures
Selecting the right data structure for your application is essential for optimal performance. Consider the complexity and requirements of your application when choosing the appropriate data structure. For example, using a list in Python when a set would be more efficient can lead to performance bottlenecks.
Take a look at this example:
def inefficient_function(numbers, target):
for number in numbers:
if target - number in numbers:
return True
return False
def efficient_function(numbers, target):
numbers_set = set(numbers)
for number in numbers_set:
if target - number in numbers_set:
return True
return False
In the above example, the efficient_function
uses a set instead of a list, reducing the time complexity and improving performance.
3. Optimize loops
Loops are a common source of performance bottlenecks. To optimize them, consider the following strategies:
- Use
for
loops instead ofwhile
loops when possible, as they are generally faster and more readable. - Minimize loop overhead by moving computations and function calls outside the loop.
- Unroll loops when the number of iterations is fixed and small, to reduce loop overhead.
Consider this example:
def calculate_squares(numbers):
result = []
for number in numbers:
result.append(number ** 2)
return result
By using a list comprehension, we can optimize the loop and improve performance:
def calculate_squares(numbers):
return [number ** 2 for number in numbers]
4. Use efficient algorithms
Selecting an efficient algorithm can greatly impact your code’s performance. Always consider the time complexity of the algorithm and choose the one that best suits your application’s requirements. For instance, using bubble sort when quicksort or merge sort would be more efficient can lead to performance issues.
For a comprehensive resource on algorithms, check out our Mastering JavaScript: A Comprehensive Guide to DOM Manipulation and Event Handling for Interactive Websites.
5. Utilize caching
Caching can significantly improve performance by storing the results of expensive computations and reusing them when needed. This reduces the need to recompute data, saving both time and resources. There are various caching techniques, such as memoization, that can be used depending on your specific use case.
Here’s an example of using memoization in a Python function:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
In the above example, the @lru_cache
decorator caches the results of the fibonacci
function, improving its performance.
6. Optimize function calls
Function calls can be expensive in terms of performance. To optimize them, consider the following strategies:
- Inline small functions to reduce function call overhead.
- Use built-in functions when possible, as they are typically faster than user-defined functions.
- Avoid using recursion for large input sizes, as it can lead to a high number of function calls and stack overflow issues.
For example, instead of using a recursive factorial function:
def factorial(n):
if n == 0:
return 1
return n * factorial(n-1)
Use an iterative approach:
def factorial(n):
result = 1
for i in range(1, n+1):
result *= i
return result
7. Minimize I/O operations
I/O operations, such as reading from or writing to a file, can be slow and negatively impact performance. To optimize I/O operations, consider the following tips:
- Use buffering to reduce the number of I/O operations.
- Read or write large chunks of data at once instead of small pieces.
- Close files as soon as they are no longer needed to free up resources.
For more on I/O operations in Python, refer to our How to Split a List in Python: A Comprehensive Guide.
8. Use lazy evaluation
Lazy evaluation is a technique where an expression is only evaluated when its value is needed. This can improve performance by avoiding unnecessary calculations. In Python, you can use generators to implement lazy evaluation. Here’s an example:
def even_numbers(numbers):
for number in numbers:
if number % 2 == 0:
yield number
numbers = range(1, 11)
even_numbers_generator = even_numbers(numbers)
for even_number in even_numbers_generator:
print(even_number)
In the above example, the even_numbers
function uses a generator to yield even numbers one at a time, avoiding the need to store all even numbers in memory. This can lead to significant performance improvements when dealing with large datasets.
9. Parallelize tasks
Running tasks in parallel can significantly improve performance by utilizing multiple CPU cores or threads. In Python, you can use the concurrent.futures
module to parallelize tasks easily. Here’s an example of parallelizing a simple function:
import concurrent.futures
def square(x):
return x * x
numbers = range(1, 11)
results = []
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = [executor.submit(square, number) for number in numbers]
for future in concurrent.futures.as_completed(futures):
results.append(future.result())
print(results)
In the example above, the square
function is executed in parallel for each number in the numbers
list, resulting in a performance boost.
10. Profile and optimize code
Profiling your code can help you identify performance bottlenecks and optimize them. There are various profiling tools available, such as Python’s built-in cProfile
module. After profiling your code, you can use various optimization techniques, such as algorithmic optimization, memory optimization, and code refactoring, to improve performance.
For more information on profiling and optimization, check out our guide on The Ultimate Guide to API Integration: Connecting Your App with RESTful Services Using Postman and Swagger.
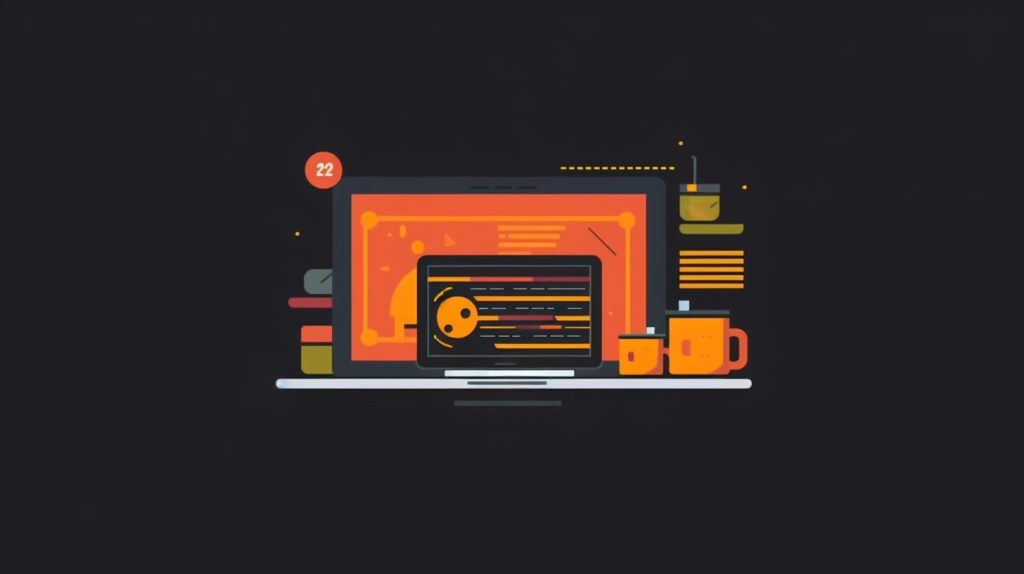
11. Use caching to reduce redundant computations
Caching is a technique where you store the results of expensive computations and reuse them when needed, instead of recalculating the same results repeatedly. This can be particularly useful in situations where you are dealing with repetitive data or calculations. In Python, you can use the functools.lru_cache
decorator to enable caching for a function:
import functools
@functools.lru_cache(maxsize=None)
def fib(n):
if n < 2:
return n
return fib(n - 1) + fib(n - 2)
print(fib(100))
In this example, the Fibonacci function is decorated with the lru_cache
decorator, which caches the results of the function calls. This significantly speeds up the execution of the function, especially for larger input values.
12. Use appropriate data structures
Choosing the right data structure for your problem can have a significant impact on performance. For example, using a set instead of a list for membership testing can speed up your code because sets use hashing for membership tests, while lists require linear search. Similarly, using a deque (double-ended queue) from the collections
module can be more efficient for certain operations than using a list.
For more information on data structures in Python, refer to our Powerful Python Tips: Web Scraping article.
13. Use vectorized operations with NumPy
When working with numerical data, using vectorized operations with NumPy can lead to significant performance improvements. Vectorized operations are implemented in C or Fortran and can take advantage of low-level optimizations and parallelism. Here’s an example of a vectorized addition operation:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([5, 4, 3, 2, 1])
result = a + b
print(result)
In this example, the addition operation is performed element-wise on the two NumPy arrays, resulting in much faster execution compared to using Python’s built-in lists and loops.
14. Use the right libraries
Many libraries have been developed to optimize specific operations or algorithms, so it’s essential to choose the right library for your task. For example, when working with large datasets, using libraries like Pandas or Dask can lead to better performance than using pure Python code. Similarly, for machine learning tasks, using libraries like TensorFlow or PyTorch can provide significant performance improvements over custom implementations.
15. Use JIT compilation
Just-In-Time (JIT) compilation is a technique that compiles your code at runtime, which can lead to significant performance improvements. Some libraries, like Numba and PyPy, offer JIT compilation for Python code. Here’s an example of using Numba’s JIT compiler:
import numba
@numba.jit(nopython=True)
def add_arrays(a, b):
result = np.empty(a.shape)
for i in range(a.shape[0]):
result[i] = a[i] + b[i]
return result
a = np.random.rand(1000000)
b = np.random.rand(1000000)
result = add_arrays(a, b)
print(result)
In this example, the add_arrays
function is decorated with Numba’s jit
decorator, which compiles the function at runtime, leading to faster execution.
16. Use parallelism and concurrency
When working with tasks that can be executed independently, using parallelism or concurrency can significantly improve performance. In Python, you can use the concurrent.futures
library to parallelize your code using threads or processes. Here’s an example of using the ThreadPoolExecutor
for parallel execution:
from concurrent.futures import ThreadPoolExecutor
import time
def slow_function(x):
time.sleep(1)
return x * 2
with ThreadPoolExecutor(max_workers=5) as executor:
results = list(executor.map(slow_function, range(5)))
print(results)
In this example, the slow_function
is executed in parallel using a thread pool, reducing the overall execution time.
17. Profile your code
Profiling your code is crucial to identify performance bottlenecks and optimize them effectively. Python provides built-in tools, like cProfile
and timeit
, to measure the execution time of your code and identify slow functions. Additionally, you can use third-party tools like Py-Spy or Pyflame for more advanced profiling.
For more information on profiling your code, refer to our Mastering JavaScript: A Comprehensive Guide to DOM Manipulation and Event Handling for Interactive Websites article.
18. Optimize memory usage
Reducing memory usage can improve the performance of your code, especially in memory-bound applications. You can use Python’s built-in memory_profiler
module or third-party tools like Pympler to measure memory usage and identify memory leaks. Additionally, you can use libraries like NumPy or Pandas to store and manipulate large datasets more efficiently.
19. Use compiled languages for performance-critical code
If you have performance-critical code that cannot be optimized further in Python, consider rewriting that part of the code in a compiled language like C, C++, or Rust. You can then use tools like Cython or CFFI to interface with the compiled code from Python. This can result in significant performance improvements for specific tasks.
20. Optimize I/O operations
I/O operations, like reading and writing to disk or network, can be a significant performance bottleneck in your code. To optimize I/O operations, consider using asynchronous I/O with libraries like asyncio
or using buffered I/O with the io
module. Additionally, use efficient file formats like Parquet or Avro for large datasets to minimize I/O overhead.
21. Use profiling and monitoring tools
Profiling and monitoring tools can help you identify performance bottlenecks and optimize your code effectively. Some popular tools include Py-Spy, Pyflame, and the built-in cProfile
module. You can also use monitoring tools like Datadog or Prometheus to monitor the performance of your applications in production environments.
22. Optimize database queries
When working with databases, optimizing your queries can have a significant impact on performance. Some common techniques for optimizing database queries include indexing, query optimization, and denormalization. You can also use Object-Relational Mapping (ORM) libraries like SQLAlchemy or Django ORM to help optimize your database interactions.
23. Use connection pooling for databases
Opening and closing database connections can be an expensive operation, leading to performance bottlenecks. Using connection pooling can help alleviate this issue by reusing existing connections instead of creating new ones. Most database drivers and ORM libraries, like psycopg2 for PostgreSQL or SQLAlchemy, support connection pooling out-of-the-box.
24. Use HTTP caching for web applications
If you’re building a web application, using HTTP caching can significantly improve performance by reducing the need for repeated requests to your server. There are several types of HTTP caching, including browser caching, server-side caching, and Content Delivery Network (CDN) caching. Implementing the appropriate caching strategy can lead to a faster and more responsive web application.
25. Optimize server and deployment settings
Last but not least, optimizing your server and deployment settings can help improve the performance of your Python applications. Some settings to consider include using a production-ready web server (e.g., Gunicorn or uWSGI), fine-tuning worker processes and threads, and using reverse proxies like Nginx or Apache for load balancing and SSL termination. Additionally, ensure that you’re using the latest versions of Python, libraries, and operating systems for optimal performance.
In conclusion, optimizing Python code can be achieved through various techniques and tools. By following the tips mentioned above, you can make your Python applications more efficient, faster, and more scalable. Always remember to profile and monitor your code to identify bottlenecks and implement the appropriate optimizations for your specific use case.